SQL Subqueries
Getting Started with SQL Subqueries: Beginner's Guide
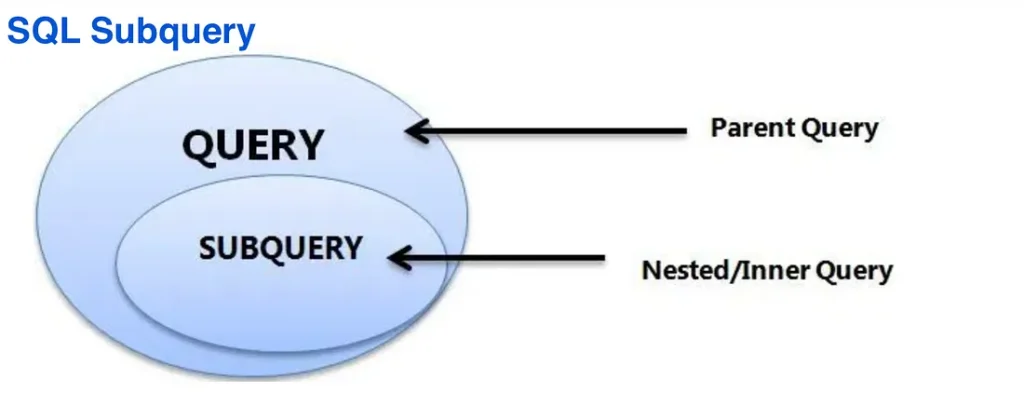
Structured Query Language (SQL) is like a magic wand for managing and playing with data in databases. It can do more than just simple searches and joins. In this article, we will break down the complexities of SQL subqueries, making them more accessible and demonstrating how they can empower you to uncover valuable insights within your data. Whether you’re new to SQL or an experienced practitioner, join us as we explore the fascinating world of subqueries and discover how they can elevate your data analysis skills.”
Subqueries: Your Data Detective
A subquery is like a secret agent query inside another query. It’s a special tool that lets you use the results of one query in another. You can use subqueries in different parts of your SQL command, like when you want to:
Filter Rows: Imagine you have a list of customers and their orders. Subqueries can help you find customers who’ve placed orders. It’s like saying, “Show me all the customers who are also in the orders list.
SELECT customer_name FROM customers WHERE customer_id IN (SELECT customer_id FROM orders);
In this example, the subquery finds all the customer IDs from the “orders” table, and the main query picks out customers who match those IDs in the “customers” table. This helps you find customers who’ve ordered something.
Math and Aggregates: Subqueries can also help you do math with data. Let’s say you want to find employees whose salary is higher than the average in their department. It’s like finding the top earners in each team.
SELECT employee_name, salary FROM employees WHERE salary > (SELECT AVG(salary) FROM employees WHERE department_id = employees.department_id);
In this case, the subquery calculates the average salary for each department, and the main query finds employees whose salary beats that average. This helps you spot high earners in each team.
Linked Subqueries: Some subqueries even talk to the main query. They look at what the main query is doing and adjust their results. For example:
SELECT product_name FROM products p WHERE price > (SELECT AVG(price) FROM products WHERE category_id = p.category_id);
Here, the subquery calculates the average price for each product category, and the main query picks products with prices higher than the average for their category. It’s like finding the expensive items in each group.
Derived Tables: Your Temporary Data Playground
A derived table is like a pop-up table that appears just when you need it. It’s temporary and created within your SQL command. This table is useful when you want to do some fancy stuff with your data, like making it dance or mix it up.
Here’s a simple example:
SELECT d.department_name, e.total_salary FROM ( SELECT department_id, SUM(salary) AS total_salary FROM employees GROUP BY department_id ) AS e JOIN departments d ON e.department_id = d.department_id;
In this query, the derived table (we call it “e”) calculates the total salary for each department. Then, the main query joins this “e” table with the “departments” table to get department names and total salaries. It’s like making a report card for each department.
Why Use These Tricks?
- Simplify Complex Problems: Subqueries and derived tables help you break big problems into smaller, easier ones in sql
- Reuse Your Code: You can save your tricks and use them again and again. It’s like having your favorite magic spells ready.
- Speed Things Up: These tricks can make your searches faster by sorting and filtering data before it gets complicated.
- Get Creative: You can do all sorts of cool stuff, like math, filtering, and transforming data, right inside your SQL command.
- Make Reporting Easy: When you’re making reports or doing fancy analysis, these tricks help you get the data you need without pulling your hair out.
But beware! Too many tricks can slow things down. Here are some tips:
- Optimize: Make sure your tricks are well-oiled. Use the right tools, don’t pull too much data, and structure your code smartly.
- Don’t Overdo It: Use these tricks wisely. Too many tricks can make your code messy and hard to understand.
- Index Your Data: Make sure the columns you’re using in your tricks are properly organized. This speeds up your magic
- Test and Fix: Always check how your tricks perform with different data. Fix any problems you find.
In summary, subqueries and derived tables are like hidden gems in SQL. They help you solve complex problems, make your code reusable, and speed up your searches. Just remember to use them wisely and keep your magic wand (your SQL skills) well-practiced. You’ll become a data wizard in no time!